Dynamic number insertion for call tracking
Learn to display different phone numbers in your website according to your traffic source
Learn how to measure the effectiveness of online campaigns and track the amount of calls generated after someone visits your website from any of the following means of online marketing:
- Direct traffic to your website
- Organic traffic from search engines
- Facebook post
- Twitter posts
- Campaigns in Google AdWords
- Campaigns in AdRoll
The following code will allow the phone numbers of your website to change dynamically depending on the traffic source, and we are going to need some javascript code for this.
Get the phone numbers to assign to each traffic source
You must buy the virtual phone numbers you want to use. You can assign a friendly name to each one to differentiate it later in the calls report.
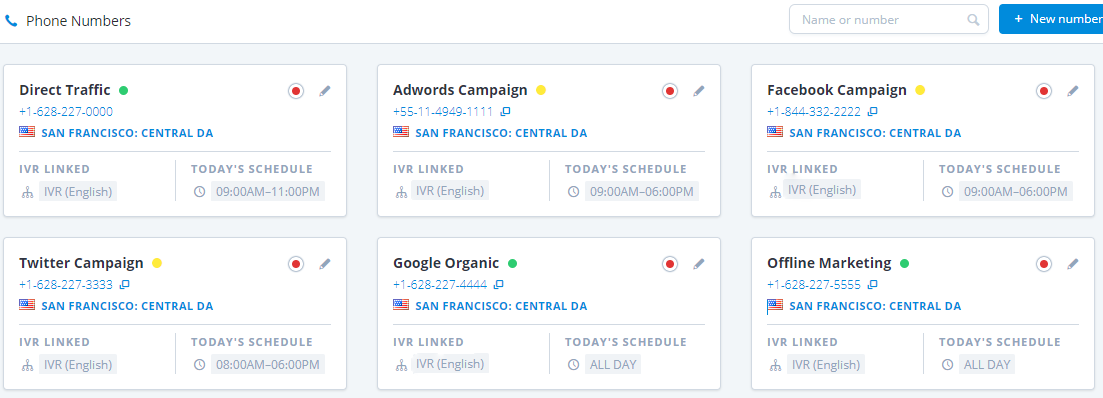
Call tracking phone numbers
Checking call conversions
You can use the Calls per number report to see how many calls were received for every phone number and you can validate which digital campaign is more successful.
Preparing the website to change phone numbers
You must add the CSS Class businessPhoneNumber to each HTML element that will display a phone number in your website.
<div class="container">
<h1>Welcome to my Website!</h1>
<p>Phone number in a span:<span class="businessPhoneNumber">123456789</span></p>
Phone number in a link:
<a class="businessPhoneNumber" href="tel:+18443326433"> +1 844 332 6433 </a>
</div>
Adding the javascript code to dynamic number insertion
We will use the library Sourcebuster.js to identify the traffic source of the visitor and use it so you can show the phone number depending on the source of web traffic. You can download this library or use a CDN as we will do in the example.
We have to modify the changePhoneNumberPage function to add the different phone numbers depending on each of the traffic sources detected by SourceBuster. There are different blocks of code like the following where we will replace FACEBOOK SPECIFIC PHONE NUMBER with the phone number we want to display when the visitor comes from Facebook for example.
//Detect social phone number source
if(trafficSource.src=="facebook.com"){
changePhoneNumber(phoneNumberElement,"FACEBOOK SPECIFIC PHONE NUMBER");
};
Repeat the process for each traffic source; if you want to avoid changing the phone number for any traffic source, just put that line in comment:
if(trafficSource.src=="twitter.com"){
// changePhoneNumber(phoneNumberElement,"TWITTER SPECIFIC PHONE NUMBER");
}
Full code to insert in your website
This is the complete block of code that you can copy and paste into your website.
<!--Add the reference to sourcebuster javascript library-->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/sourcebuster.min.js"></script>
<script>
//Configure initial parmeters
sbjs.init({
session_length: 5, //session of minutes. You can change it if you need more
referrals: [ //Configure social medium
{
host: 'l.facebook.com',
medium: 'social',
display: 'facebook.com'
},
{
host: 'web.facebook.com',
medium: 'social',
display: 'facebook.com'
},
{
host: 'l.messenger.com',
medium: 'social',
display: 'facebook.com'
},
{
host: 't.co',
medium: 'social',
display: 'twitter.com'
}
]
});
//Change the phone number depending on the traffic source
changePhoneNumberPage(sbjs.get.current,"businessPhoneNumber");
//trafficSource: sourcebuster object with all the session data
//numberClassName: CSS class that identify the html elements to change dynamically the phone number
function changePhoneNumberPage(trafficSource,numberClassName){
//Get an array of elements to change
var phoneNumberElement = document.getElementsByClassName(numberClassName);
//Add the direct traffic phone, this is the default phone number
changePhoneNumber(phoneNumberElement,"DEFAULT PHONE");
//Detect social phone number source
if(trafficSource.src=="facebook.com"){
changePhoneNumber(phoneNumberElement,"FACEBOOK SPECIFIC PHONE NUMBER");
};
if(trafficSource.src=="twitter.com"){
changePhoneNumber(phoneNumberElement,"TWITTER SPECIFIC PHONE NUMBER");
}
//Detect Google Ads traffic
if(trafficSource.src=="google" && trafficSource.mdm=="cpc"){
changePhoneNumber(phoneNumberElement,"ADWORDS SPECIFIC PHONE NUMBER");
}
//Detect Adroll traffic
if(trafficSource.src=="adroll"){
changePhoneNumber(phoneNumberElement,"ADROLL SPECIFIC PHONE NUMBER");
}
//Detect Google organic traffic
if(trafficSource.src=="google" && trafficSource.mdm=="organic"){
changePhoneNumber(phoneNumberElement,"GOOGLE ORGANIC PHONE NUMBER");
}
//Detect other organic traffic: Bing, Yandex, Yahoo etc.
if(trafficSource.src!="google" && trafficSource.mdm=="organic"){
changePhoneNumber(phoneNumberElement,"OTHER ORGANIC PHONE NUMBER");
}
};
//phoneNumberElement: array of elements with de CSS class name
//phoneNumber: Phone number to change
function changePhoneNumber(phoneNumberElement,phoneNumber){
for (var i = 0; i < phoneNumberElement.length; i++) {
//Change the text in every element by the phone number
phoneNumberElement[i].innerText=phoneNumber;
//If the element is a link element we add the hyperlink with tel parameter
if (phoneNumberElement[i].localName=="a"){
phoneNumberElement[i].href="tel:+"+phoneNumber;
}
}
};
</script>
About Sourcebuster options
SourceBuster has several options that maybe you or your development team can use. This library stores cookies to register user visits and manage both the first and recent visits. Learn more options from this library on their website
The following code in JSFiddle can be useful to you and it will show you the code working.
Updated about 5 years ago